A ConfigMap is provided as a way to inject configuration data into a pod/pods. It can be included as files or be used as environment variables. Below are a couple of examples of mounting a configmap using both methods.
Use as File
In this first example I’ll show how to create a configmap and mount it using the key(s) as filenames and the data as the file content. It will then be used in an Nginx container as the index page.
First create the configmap if the pod is created first it won’t start properly as it wouldn’t be able to find the configmap.
# file: nginx-cm.yaml
apiVersion: v1
kind: ConfigMap
metadata:
name: nginx-cm
data:
index.html: Hello, World!
You can check if the configmap is available using kubectl get configmaps
. The output should show nginx-cm
.
Next create the pod, once it is up and running create the service and view the page in the browser. It should appear plain white with the only words being “Hello, World!”.
# file: nginx-pod.yaml
apiVersion: v1
kind: Pod
metadata:
name: nginx
spec:
containers:
- image: nginx:alpine
imagePullPolicy: IfNotPresent
name: nginx
volumeMounts:
- name: nginx-files
mountPath: /usr/share/nginx/html
readOnly: true
volumes:
- name: nginx-files
configMap:
name: nginx-cm
# file: nginx-service.yaml
apiVersion: v1
kind: Service
metadata:
creationTimestamp: null
labels:
run: nginx
name: nginx
spec:
ports:
- port: 80
protocol: TCP
targetPort: 80
nodePort: 30080
selector:
run: nginx
type: NodePort
status:
loadBalancer: {}
Use as Environment Variable
Now let’s take a look at using the configmap as environment variables. In this example I’ll use the Kode Kloud webapp color so the value and any changes will be easily visible.
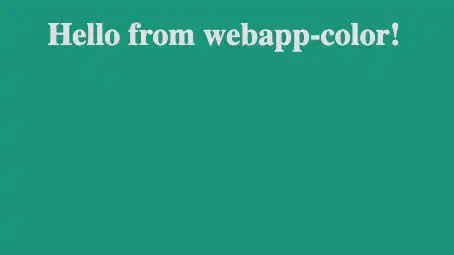
Like last time the first thing to do is create the configmap so it will be available to the pod. To start we’ll use the color green.
# file: webapp-color-cm.yaml
apiVersion: v1
kind: ConfigMap
metadata:
name: webapp-color-cm
data:
app-color: green
Check if the configmap is available using kubectl get configmaps
. The output should show webapp-color-cm
.
Next create the pod, I added some comments in the yaml to help point out the configmap mapping and how to choose the environment variable name.
# file: webapp-color-pod.yaml
apiVersion: v1
kind: Pod
metadata:
labels:
run: webapp-color
name: webapp-color
spec:
containers:
- name: test-container
image: kodekloud/webapp-color:latest
imagePullPolicy: IfNotPresent
env:
# the environment variable that will be set
- name: APP_COLOR
valueFrom:
configMapKeyRef:
# the name of the configmap created previously
name: webapp-color-cm
# the key to use the data from
key: app-color
Next create the service and view the resulting page. Hopefully it appears green!
# file: webapp-color-service.yaml
apiVersion: v1
kind: Service
metadata:
creationTimestamp: null
labels:
run: webapp-color
name: webapp-color
spec:
ports:
- port: 8080
protocol: TCP
targetPort: 8080
nodePort: 30080
selector:
run: webapp-color
type: NodePort
status:
loadBalancer: {}
If you would like to change the background color of the page; it’s possible to edit the configmap. You’ll have to recreate the pod for the changes to be picked up though.
kubectl edit configmaps webapp-color-cm
Change ‘green’ to ‘red’ then save and exit the editor.
Finally recreate the pod, you can either delete and then create again or run the following kubectl replace -f webapp-color-pod.yaml --force
. Once this finishes and the pod is ready again, refresh the page and the background will now be red.